Lightning Slots App
Come and play real slots and go for the Jackpot! Lightning Link Casino is the app brought to you by the makers of online slots games Heart of Vegas and Cashman Casino, Aristocrat. Get ready to win big! Flip the switch and get charged up for an electrifying new slots games journey with Lightning Link Casino. The most exciting new slot. Lightning Link Casino is the app brought to you by the makers of online slots games Heart of Vegas and Cashman Casino, Aristocrat. Get ready to win big! Flip the switch and get charged up for an.
You can add components within the body of another component. Composition enables you to build complex components from simpler building-block components. Composing apps and components from a set of smaller components makes code more reusable and maintainable.
Let’s look at a simple app. The components are in the example
namespace. The markup is contrived because we want to illustrate the concepts of owner and container.
Owner
The owner is the component that owns the template. In this example, the owner is the example-todo-app
component. The owner controls all the composed (child) components that it contains. An owner can:
- Set public properties on composed components
- Call public methods on composed components
- Listen for events dispatched by composed components
Container
A container contains other components but itself is contained within the owner component. In this example, example-todo-wrapper
is a container. A container is less powerful than the owner. A container can:
- Read, but not change, public properties in contained components
- Call public methods on composed components
- Listen for some, but not necessarily all, events bubbled up by components that it contains. See Configure Event Propagation.
Parent and child
When a component contains another component, which, in turn, can contain other components, we have a containment hierarchy. In the documentation, we sometimes talk about parent and child components. A parent component contains a child component. A parent component can be the owner or a container.
# Set a Property on a Child Component
To communicate down the containment hierarchy, an owner can set a property on a child component. An attribute in HTML turns into a property assignment in JavaScript.
Let’s look at how the owner, example-todo-app
, sets public properties on the two instances of example-todo-item
.
In the playground, select todoItem.js
. The @api
decorator exposes the itemName
field as a public property.
Select todoApp.html
. To set the public itemName
property, it sets the item-name
attribute on each example-todo-item
component. Change Milk
to Hummus
to see an item name change.
Property names in JavaScript are in camel case while HTML attribute names are in kebab case (dash-separated) to match HTML standards. In todoApp.html
, the item-name
attribute in markup maps to the itemName
JavaScript property of todoItem.js
.
Tip
This example uses the static values Milk
and Bread
. A real-world component would typically use a for:each
iteration over a collection computed in the owner’s JavaScript file, todoApp.js
.
Let's look at another example of composition and data binding. The example-contact-tile
component exposes a contact
public property (@api contact
in contactTile.js
). The parent component, example-composition-basics
, sets the value of contact
.
The contact
public property in example-contact-tile
is initialized based on the contact
property value of its owner. The data binding for property values is one way. If the property value changes in the owner, the updated value propagates to the child. For example, in compositionBasics.js
, change Name: 'Amy Taylor',
to Name: 'Stacy Adams',
. The change is reflected in the example-contact-tile
child component.
Example
See the Composition recipes in the Lightning Web Components recipes app.
# Send an Event from a Child to an Owner
When a component decorates a field with @api
to expose it as a public property, it should set the value only when it initializes the field, if at all. After the field is initialized, only the owner component should set the value.
To trigger a mutation for a property value provided by an owner component, a child component can send an event to the owner. The owner can change the property value, which propagates down to the child.
Example
The Lightning Web Components recipes app has a more realistic example. Click Child-to-Parent and look at the EventWithData example, which has a list of contacts. When a user clicks a contact, the component sends an event to the parent, which sends a new contact
object to the child.
# Data Flow Considerations
To prevent code complexity and unexpected side effects, data should flow in one direction, from owner to child.
# Primitive Property Values
We recommend using primitive data types for public properties instead of using object data types. Slice complex data structures in a higher-level component and pass the primitive values to the component descendants.
Primitive values require specific @api
properties that clearly define the data shape. Accepting an object or an array requires documentation to specify the shape. If an object shape changes, consumers break.
Standard HTML elements accept only primitive values for attributes. When a standard HTML element needs a complex shape, it uses child components. For example, a table
element uses tr
and td
elements. Only primitive types can be defined in HTML. For example, <table data={...}>
isn't a value in HTML. However, you could create a table
Lightning web component with a data
API.
# Objects Passed to Components Are Read-Only
A non-primitive value (like an object or array) passed to a component is read-only. The component cannot change the content of the object or array. If the component tries to change the content, you see an error in the browser console.
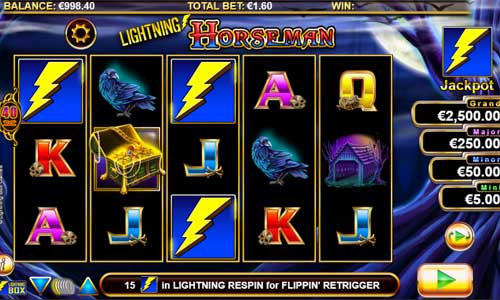
To mutate the data, the component can make a shallow copy of the objects it wants to mutate.
More commonly, the component can send an event to the owner of the object. When the owner receives the event, it mutates the data, which is sent back down to the child.
Tip
The next example attempts to help you fully understand that objects passed to a component are read-only. The example doesn't contain best practices, instead, it shows code that doesn't work.
In the following playground, example-contact-tile-object
exposes its contact
field as a public property. The parent, example-composition-basics-object
, defines a contact
object and passes it to example-contact-tile-object
. The child component can't mutate the object.
- Open the browser's JavaScript console and clear it.
- Click Update contact.name. The
contact-tile-object
component tries and fails to update thename
property of the object that it received from its owner.
The browser console displays the error: Uncaught Error: Invalid mutation: Cannot set 'name' on '[object Object]'. '[object Object]' is read-only.
The component doesn't own the object passed to it and can't mutate it.
This is a tricky but important thing to understand. The contact
object passed to example-contact-tile-object
is read-only. That object is owned by the component that passed it to the child. However, the child owns its contact
field and can set that field to a new value. Let's look at some code to see how this works.
- Click Update contact field. The
contact-tile-object
component successfully sets a new value for thecontact
field.
Again, the contact-tile-object
component owns its contact
field, and it can assign a new value to that field.
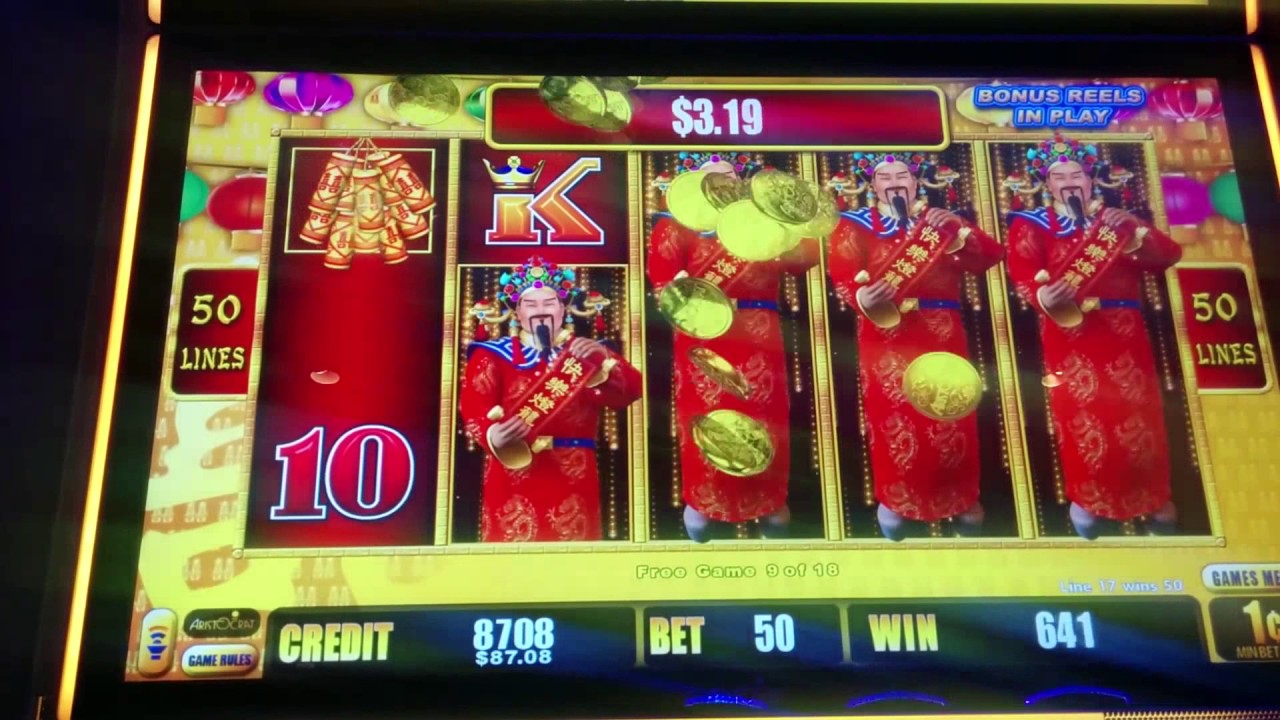
Note
After clicking Update contact field, the child component owns the object, which means that the child can mutate the object. Therefore, if you click Update contact.name again, you may expect the component to display Jennifer Wu
, but it doesn't. The value is set, and Jennifer Wu
displays in the console, which means that the component did mutate the object. However, the component doesn't rerender. Why? Because LWC can't see that a property of the contact
object changed. The contact
field is decorated with @api
, not @track
. (A field can have only one decorator.) The @api
decorator simply makes a field public and allows its value to be set from the outside. The @api
decorator doesn't tell LWC to observe mutations like @track
does.
But why does the component rerender when we assign a new value directly to the contact
field? Because LWC observes all fields for mutation. If you assign a new value to a field, the component rerenders. However, if an object is assigned to a field, LWC doesn't observe the internal properties of that object. Therefore, the component doesn't update when you assign a new value to contact.name
. To tell LWC to observe the internal properties of an object, decorate the field with @track
. See Field Reactivity.
# Call a Method on a Child Component
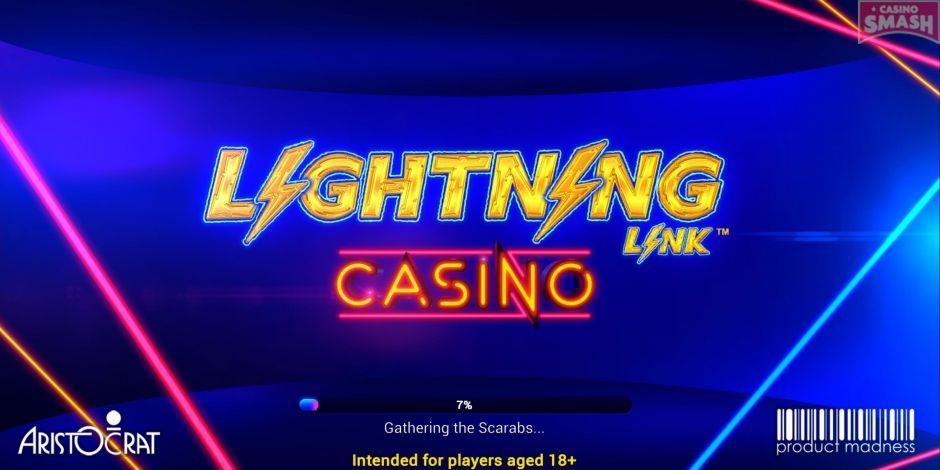
To expose a public method, decorate it with @api
. Public methods are part of a component’s API. To communicate down the containment hierarchy, owner and parent components can call JavaScript methods (or set properties) on child components.
Example
See the Parent-to-Child recipes in the Lightning Web Components recipes app.
# Define a Method
This example exposes isPlaying()
, play()
, and pause()
methods in a example-video-player
component by adding the @api
decorator to the methods. A parent component that contains example-video-player
can call these methods. Here’s the JavaScript file.
videoUrl
is a public reactive property. The @api
decorator can be used to define a public reactive property, and a public JavaScript method, on a component. Public reactive properties are another part of the component’s public API.
Note
To access elements that the template owns, the code uses the template
property.
Now, let’s look at the HTML file where the video element is defined.
In a real-world component, example-video-player
would typically have controls to play or pause the video itself. For this example to illustrate the design of a public API, the controls are in the parent component that calls the public methods.
# Call a Method
The example-method-caller
component contains example-video-player
and has buttons to call the play()
and pause()
methods in example-video-player
. Here’s the HTML.
Clicking the buttons in example-method-caller
plays or pauses the video in example-video-player
after we wire up the handlePlay
and handlePause
methods in example-method-caller
.
Here’s the JavaScript file for example-method-caller
.
The handlePlay()
function in example-method-caller
calls the play()
method in the example-video-player
element. this.template.querySelector('example-video-player')
returns the example-video-player
element in methodCaller.html. The this.template.querySelector()
call is useful to get access to a child component so that you can call a method on the component.
The handlePause()
function in example-method-caller
calls the pause()
method in the example-video-player
element.
# Return Values
To return a value from a JavaScript method, use the return
statement. For example, see the isPlaying()
method in example-video-player
.
# Method Parameters
To pass data to a JavaScript method, define one or more parameters for the method. For example, you could define the play()
method to take a speed
parameter that controls the video playback speed.
# Pass Markup into Slots
Add a slot to a component’s HTML file so a parent component can pass markup into the component. A component can have zero or more slots.
A slot is a placeholder for markup that a parent component passes into a component’s body. Slots are part of the Web Component specification.
To define a slot in markup, use the <slot>
tag, which has an optional name
attribute.
# Unnamed Slots
In the playground, click slotDemo.html
to see an unnamed slot. The slotWrapper
component passes content into the slot.
When example-slot-demo
is rendered, the unnamed slot is replaced with Content from Slot Wrapper. Here’s the rendered HTML of example-slot-wrapper
.
If a component has more than one unnamed slot, the markup passed into the body of the component is inserted into all the unnamed slots. This UI pattern is unusual. A component usually has zero or one unnamed slot.
# Named Slots
This example component has two named slots and one unnamed slot.
Here’s the markup for a parent component that uses example-named-slots
.
The example-slots-wrapper
component passes:
Willy
into thefirstName
slotWonka
into thelastName
slotChocolatier
into the unnamed slot
Here’s the rendered output.
# Run Code on slotchange
All <slot>
elements support the slotchange
event. The slotchange
event fires when a direct child of a node in a <slot>
element changes, such as when new content is appended or deleted. Only <slot>
elements support this event.
Changes within the children of the <slot>
element don’t trigger a slotchange
event.
This example contains a <slot>
element that handles the slotchange
event.
The component example-child
is passed into the slot.
The console prints the first time the component is rendered, and if the flag addOneMore
is set to true.
The slotchange
event is not triggered even when showFooter
is true and the footer element is appended.
# Query Selectors
The querySelector()
and querySelectorAll()
methods are standard DOM APIs. querySelector()
returns the first element that matches the selector. querySelectorAll()
returns an array of DOM Elements.
Call these methods differently depending on whether you want to access elements the component owns or access elements passed via slots.
Important
Don’t pass an id
to these query methods. When an HTML template renders, id
values are transformed into globally unique values. If you use an id
selector in JavaScript, it won’t match the transformed id
. If you’re iterating over an array, consider adding some other attribute to the element, like a class
or data-*
value, and use it to select the element. LWC uses id
values for accessibility only.
# Access Elements the Component Owns
To access elements rendered by your component, use the template
property to call a query method.
- The order of elements is not guaranteed.
- Elements not rendered to the DOM aren’t returned in the
querySelector()
result. - Don’t use ID selectors. The IDs that you define in HTML templates may be transformed into globally unique values when the template is rendered. If you use an ID selector in JavaScript, it won’t match the transformed ID.
Important
Don’t use the window
or document
global properties to query for DOM elements. Also, we don’t recommend using JavaScript to manipulate the DOM. It's better to use HTML directives to write declarative code.
# Access Elements Passed Via Slots
A component doesn’t own DOM elements that are passed to it via slots. These DOM elements aren’t in the component’s shadow tree. To access DOM elements passed in via slots, call this.querySelector()
and this.querySelectorAll()
. Because the component doesn't own these elements, you don’t use this.template.querySelector()
or this.template.querySelectorAll()
.
This example shows how to get the DOM elements passed to a child component from the child’s context. Pass the selector name, such as an element, to this.querySelector()
and this.querySelectorAll()
. This example passes the span
element.
# Compose Components Using Slots Versus Data
When creating components that contain other components, consider the lifecycle and construction of the component hierarchy using the declarative (slots) ors hidden from the document that contains it. The shadow tree affects how you work with the DOM, CSS, and events.
Shadow DOM is a web standard that encapsulates the elements of a component to keep styling and behavior consistent in any context. Since not all browsers implement Shadow DOM, Lightning Web Components uses a shadow DOM polyfill (@lwc/synthetic-shadow
). A polyfill is code that allows a feature to work in a web browser.
To better understand how to work with the shadow tree, let’s look at some markup. This markup contains two Lightning web components: example-todo-app
and example-todo-item
. The shadow root defines the boundary between the DOM and the shadow tree. This boundary is called the shadow boundary.
Note
The shadow root isn’t an element, it’s a document fragment.
Let’s look at how to work with the shadow tree in each of these areas.
CSS
CSS styles defined in a parent component don’t leak into a child. In our example, a p
style defined in the todoApp.css
style sheet doesn’t style the p
element in the example-todo-item
component, because the styles don’t reach into the shadow tree. See CSS.
Events

If an event bubbles up and crosses the shadow boundary, to hide the internal details of the component that dispatched the event, some property values change to match the scope of the listener. See Event Retargeting.
Access Elements
To access elements a component renders from the component’s JavaScript class, use the template
property. See Access Elements the Component Owns.
Access Slots
A slot is a placeholder for markup that a parent component passes into a component’s body. DOM elements that are passed to a component via slots aren’t owned by the component and aren’t in the component’s shadow tree. To access DOM elements passed in via slots, call this.querySelector()
and this.querySelectorAll()
. The component doesn't own these elements, so you don’t use template
. See Pass Markup into Slots.
# DOM APIs
Don’t use these DOM APIs to reach into a component’s shadow tree.
Document.prototype.getElementById
Document.prototype.querySelector
Document.prototype.querySelectorAll
Document.prototype.getElementsByClassName
Document.prototype.getElementsByTagName
Document.prototype.getElementsByTagNameNS
Document.prototype.getElementsByName
document.body.querySelector
document.body.querySelectorAll
document.body.getElementsByClassName
document.body.getElementsByTagName
document.body.getElementsByTagNameNS
Lightning Link Slots App Free Coins
Important
Lightning Slots On Facebook
The shadow DOM polyfill includes a patch to the MutationObserver
interface. If you use MutationObserver
to watch changes in a DOM tree, disconnect it or you will cause a memory leak. Note that a component can observe mutations only in its own template. It can't observe mutations within the shadow tree of other custom elements.